Figure – for loop Flowchart:
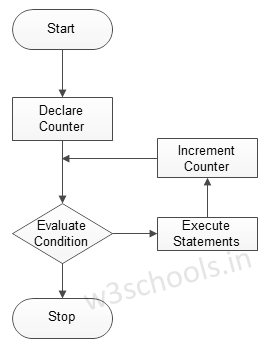
Syntax:
for iterating_var in sequence: //execute your code
Example 01:
#!/usr/bin/python
for x in range (0,3) :
print 'Loop execution %d' % (x)
Output:
Loop execution 0 Loop execution 1 Loop execution 2
Example 02:
#!/usr/bin/python
for letter in 'TutorialsCloud':
print 'Current letter is:', letter
Output:
Current letter is : T Current letter is : u Current letter is : t Current letter is : o Current letter is : r Current letter is : i Current letter is : a Current letter is : l Current letter is : s Current letter is : C Current letter is : l Current letter is : o Current letter is : u Current letter is : d
while Loop
The graphical representation of the logic behind while looping is shown below:
Figure – while loop Flowchart:
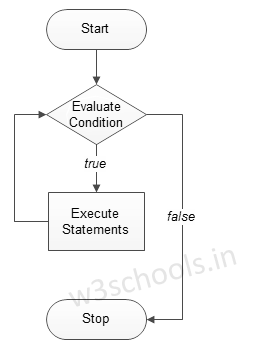
Syntax:
while expression: //execute your code
Example:
#!/usr/bin/python
#initialize count variable to 1
count =1
while count < 6 :
print count
count+=1
#the above line means count = count + 1
Output:
1 2 3 4 5
Nested Loops
Syntax:
for iterating_var in sequence: for iterating_var in sequence: //execute your code //execute your code
Example:
for g in range(1, 6):
for k in range(1, 3):
print '%d * %d = %d' % ( g, k, g*k)
Output:
1 * 1 = 1 1 * 2 = 2 2 * 1 = 2 2 * 2 = 4 3 * 1 = 3 3 * 2 = 6 4 * 1 = 4 4 * 2 = 8 5 * 1 = 5 5 * 2 = 10
0 Comments
If you have any doubts,please let me know